Similarly to the Seek node in which we set the playback position of a sound, we can create a new custom blueprint node that allows us to easily track the actual playback position of a sound in milliseconds. This can be useful for multiple purposes. For example, it can be nice to spawn and keep audio sources in sync without using the multi positional mode (allowing to affect the individual sources).
To make this happen, define a new function named GetSourcePlayPosition
in the AkGameplayStatics header file.
UFUNCTION(BlueprintCallable, BlueprintCosmetic, Category = "Audiokinetic")
static int32 GetSourcePlayPosition(int32 PlayingID);
In AkGamePlayStatics.cpp, implement the function as following:
int32 UAkGameplayStatics::GetSourcePlayPosition(int32 PlayingID)
{
AkTimeMs Position = 0;
auto Result = AK::SoundEngine::GetSourcePlayPosition((AkPlayingID)PlayingID, &Position);
if (Result != AKRESULT::AK_Success)
{
UE_LOG(LogScript, Warning, TEXT("UAkGameplayStatics::GetSourcePlayPosition: Failed"));
return 0;
}
else return (int32)Position;
}
Here we are passing our PlayingID
to AK::SoundEngine::GetSourcePlayPosition
and assigning the resulting Position
(in milliseconds) to the declared AkTimeMs
. Finally we check if the function returns AK_Success
. If yes we return the source position.
By compiling the project we get the new Get Source Play Position node. To use the node connect the returned Playing ID of a Post Ak Event node to the Get Source Play Position input pin. Here you can see a minimal example:
But there is a catch! The blueprint node won’t work as expected because we need to pass the AK_EnableGetSourcePlayPosition
flag to AK::SoundEngine::PostEvent
as mentioned in the GetSourcePlayPosition
documentation page.
To make this work for blueprints open up the AkGamePlayTypes header file and add this value to the EAkCallbackType
enum:
EnableGetSourcePlayPosition = 20 UMETA(ToolTip = "Enable AK::SoundEngine::GetSourcePlayPosition"),
And this to the static assert checks direct below:
CHECK_CALLBACK_TYPE_VALUE(EnableGetSourcePlayPosition);
Now you’ll be able to select Enable Get Source Play Position in the Callback Mask option of a Post Ak Event node:
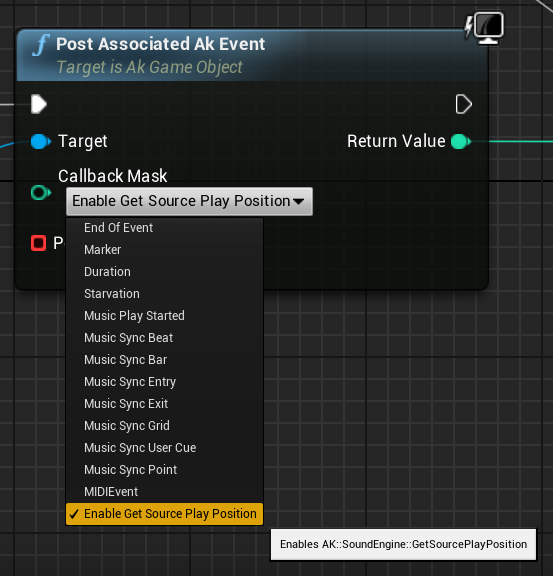
Getting the playback position in milliseconds will work now as expected:
