In this tutorial we will control FMOD Game Parameters by using Blueprint nodes and by writing simple C++ code.
Preparation in FMOD Studio
Create a new Event in FMOD Studio and insert a Continuous Parameter named Pitch with values that range between -12 and 12 (map it accordingly to the master pitch setting):

Also create a Global Parameter named EQ Global with values that range between 0 and 1. Add an automation curve, so that the frequency of the filter goes down when reaching the value 1:
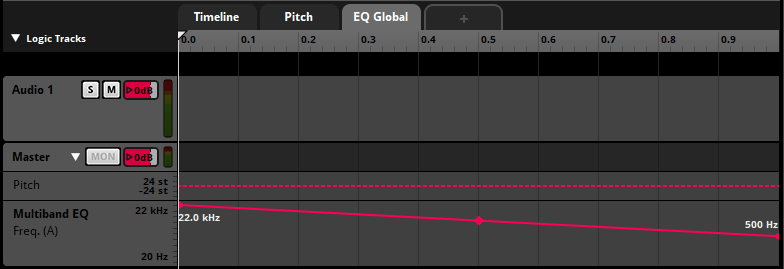
Build the banks and switch over to the Unreal Editor.
Controlling FMOD Parameters by using Blueprints
We can use the Event Instance Set Parameter node to control a Parameter of an Event Instance in Blueprints:
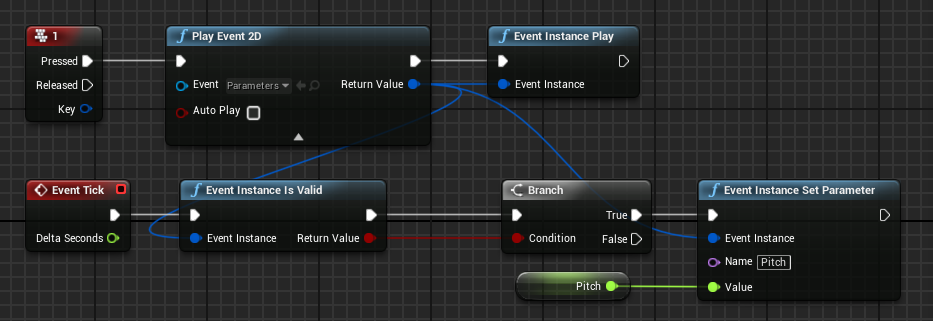
Here we are playing the Event by pressing the 1 key. We check every frame if the Event Instance is valid, and if it is, we set the Parameter to a new pitch variable (remember to make the variable instance editable). You will be able to modify the parameter value in the details tab of the Actor when playing the game.
If you are using the FMOD Ambient Sound Actor or the FMODAudio component instead, you can simply reference the FMODAudio component and use the Set Parameter node:
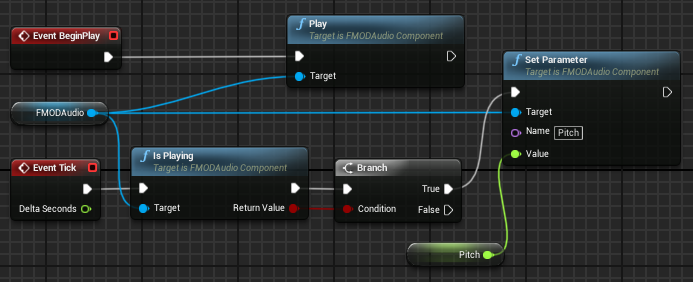
Controlling FMOD Global Parameters by using Blueprints
Use the Set Global Parameter By Name node to control Global Parameters in a Blueprint:

We extended the previous Blueprint to include the Set Global Parameter by Name node and created a new float variable named EQ that we attached to the Value field. Start the level and change the values in the Details tab of your Character/Actor
Controlling FMOD Parameters in UE4 by writing C++ code
In this tutorial section, we will take a look at how to control FMOD Parameters by interfacing with the Blueprint functions or directly accessing FMOD’s Studio API.
EventInstanceSetParameter and setParameterByName
Declare a float variable called pitch:
UPROPERTY(EditAnywhere, Category = "FMOD", meta = (ClampMin = "-12", ClampMax = "12"))
float Pitch;
The pitch variable will show up in the Details tab of our Actor thanks to the EditAnywhere specifier. ClampMin and ClampMax allow us to visually restrict the range of the variable value:
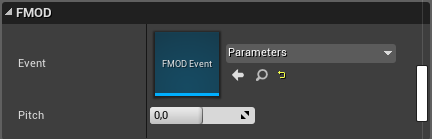
We can now call EventInstanceSetParameter
to set the Parameter to our Pitch variable:
if (UFMODBlueprintStatics::EventInstanceIsValid(InstanceWrapper))
{
UFMODBlueprintStatics::EventInstanceSetParameter(InstanceWrapper, FName("Pitch"), Pitch);
}
Alternatively, it is possible to directly change the Parameter by using FMOD’s Studio API:
InstanceWrapper.Instance->setParameterByName(TCHAR_TO_UTF8(*FName("Pitch").ToString()), Pitch);
setParameterByID
In the Character’s header file, declare a FMOD_STUDIO_PARAMETER_ID
variable:
FMOD_STUDIO_PARAMETER_ID PitchParameterId;
We can retrieve the Parameter Id by getting the Event Description of the Event Instance, after that declaring a Parameter Description variable and getting the Parameter Description by calling the getParameterDescriptionByName
function. We assign the Id of the Parameter Description to the Parameter Id variable we declared in the header file:
FMOD::Studio::EventDescription* eD;
InstanceWrapper.Instance->getDescription(&eD);
FMOD_STUDIO_PARAMETER_DESCRIPTION pD;
eD->getParameterDescriptionByName(TCHAR_TO_UTF8(*FString("Pitch")), &pD);
PitchParameterId = pD.id;
Alternatively, we can use the IFMODStudioModule singleton to get the Event Description from an UFMODEvent:
FMOD::Studio::EventDescription* eD = IFMODStudioModule::Get().GetEventDescription(Event, EFMODSystemContext::Max);
FMOD_STUDIO_PARAMETER_DESCRIPTION pD;
eD->getParameterDescriptionByName(TCHAR_TO_UTF8(*FString("Pitch")), &pD);
PitchParameterId = pD.id;
Setting a FMOD Global Parameter in C++
Let’s create a new float variable in our header file first:
UPROPERTY(EditAnywhere, Category = "FMOD", meta = (ClampMin = "0", ClampMax = "1"))
float EQ;
This variable will also be visible in the Editor. After that, we can set a Global Parameter by calling SetGlobalParameterByName
in the Character’s class:
UFMODBlueprintStatics::SetGlobalParameterByName(FName("EQ Global"), EQ);
Insert the code above in the Tick function and start the game. By moving the EQ slider in the Character’s Detail tab, you will hear the parameter change taking place.
Congratulations! You learned how to control FMOD Parameters in Unreal Engine 4. In the next tutorial we will take a look at FMOD’s Mixer.