In this tutorial we will learn how to implement multi-surface footsteps for a Third and First Person Character using FMOD in Unreal Engine 4.
Footsteps setup in FMOD Studio
In FMOD Studio, create a new Event with a Labeled Parameter named Terrain. We want to play different sounds for different floor surfaces:

The labeled parameter Terrain contains 4 entries, in this case Grass, Gravel, Wood and Water. We place a multi-instrument that contains individual samples for the terrain type at each of these values.
Footsteps Setup in Unreal Engine 4
The first thing we want to do is to set up new Physical Surfaces in Unreal’s project settings. Go to Edit->Project Settings and select the Physics tab. In the Surface Type fields, enter your footsteps terrain types:
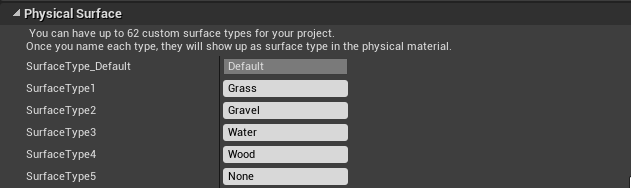
Create a new Material and a new Physical Material for each Surface Type:

Change the Surface Type in the Physical Properties settings of each Physical Material:

After that, open each Material and select the appropriate Physical Material in the Details tab:

Create a new simple level consisting of four floors and assign a material to each floor:

Implementing Footsteps for a Third Person Character with FMOD in Unreal Engine 4
Open the run or walk animation of your character and insert a new Animation Notifier at the keyframes in which your character’s feet touch the ground:

In the animation blueprint of your character, create a new reference to the ThirdPersonCharacter first by using the Cast To ThirdPersonCharacter node. Also create a new float variable named Terrain:
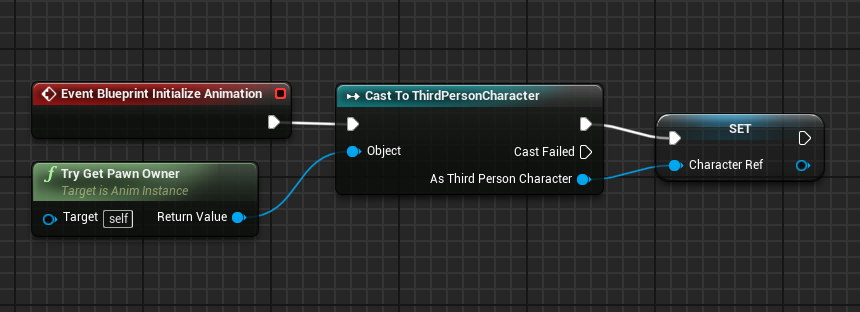
We want to cast a ray every time the Footstep Notify fires an event. For this we use the LineTraceByChannel node and connect it to the AnimNotify_Footstep node. We get the socket location of our root bone by using the Get Socket Location node and assign it to the Start vector. For the End vector, we use the Vector – Vector node and subtract (0, 0, 100) from the socket location, so that the ray fires in the direction of the character’s feet. We add the character reference to an Array and connect it to the Actors To Ignore field. After that we use the Break Hit Result node to get information about what the ray actually hit. We also check if the ray hit anything using the Branch node:
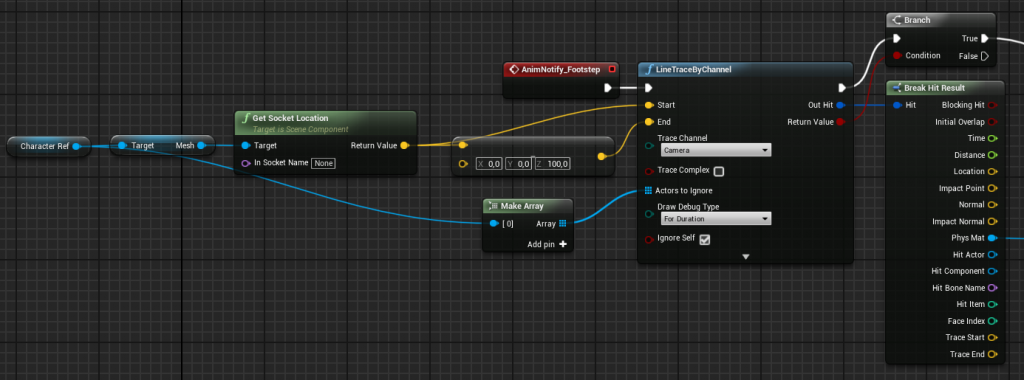
If the ray hit anything, we create the Switch on EPhysicalSurface node and connect it to the Phys Mat property of the Break Hit Result node. Thanks to the switch node, we can set the value of our Terrain variable before playing the FMOD Event. We will use the Play Event Attached node in combination with the Set Parameter and Play node to play the footstep sound. Make sure to set Auto Play on the Play Event Attached node to false, insert the parameter name into the Name property of the Set Parameter node, and connect the terrain value to the Value property of the Set Parameter node:
But how do we know that the int values correspond to certain ground types? In FMOD Studio, these values are displayed to the right of the label in the game parameter configuration:
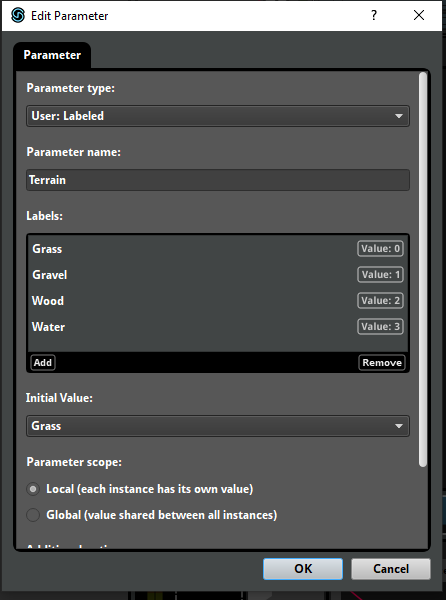
Implementing Footsteps for a First Person Character with FMOD in Unreal Engine 4
If we are working on a simple First Person Character, it may be the case that our character doesn’t have any animations in which we can add Animation Notifications. We can still implement footsteps sounds in this situation by checking if the player is moving and playing the sounds on an interval of time.
In the FirstPersonCharacter Blueprint, create a new boolean variable named isMoving. Get the velocity of the Actor by using the Get Velocity node and check if the Vector Lenght of the returned velocity is greater than 150. If that is the case, set isMoving to true and use the Set Timer by Event node to fire the custom event PlayFootstep. Make sure to check the Looping option and set the time to something between 0.3 and 0.8 seconds. Create an additional Branch node that sets the moving variable to false and clears the timer if the velocity is less then 150. Connect the two Branch nodes to the Event Tick node and to a Branch node that checks for isMoving as seen here (click to open in a new window):
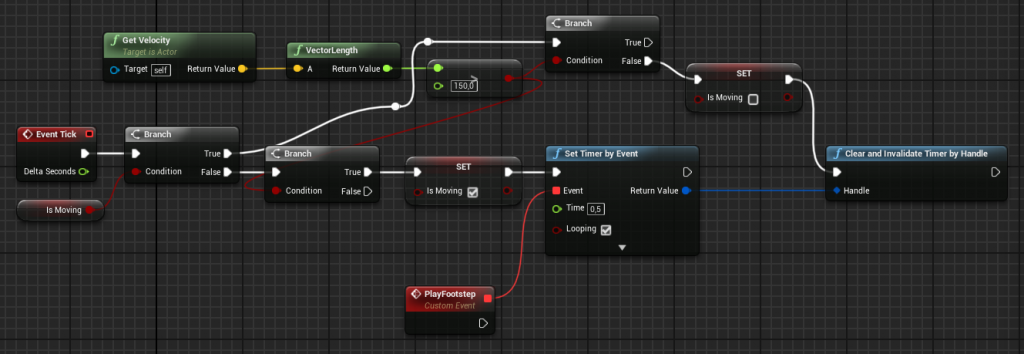
Essentially, we can copy most of the previous implementation to this Blueprint and just connect the custom PlayFootstep event to the LineTraceByChannel node:
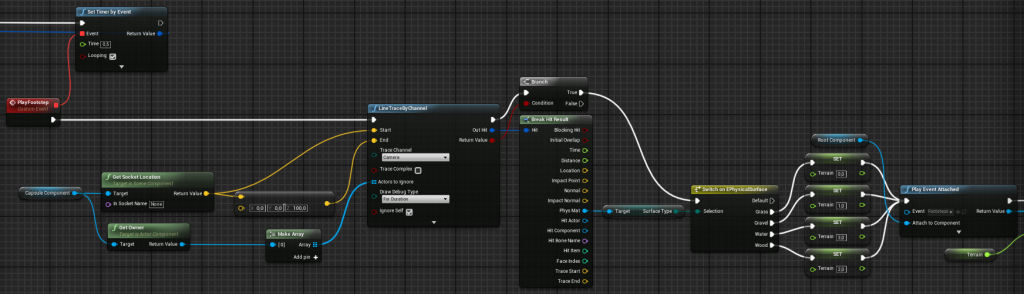
That’s it! Start the level and you should be able to hear the footsteps sound playing based on the terrain you walk on.