With parameters you can make changes to your Events and Snapshots in realtime using game variables. Whether we want to change our music dynamically or non-linearly link sounds to certain variables: the use of parameters is fun!
Setting up Parameters in FMOD Studio
Each Event in FMOD Studio begins with a parameter called Timeline.
The timeline has the special feature of progressing automatically: As long as the event is playing, the value of the timeline parameter increases continuously. Imagine the typical timeline of a DAW that goes linearly from left to right:
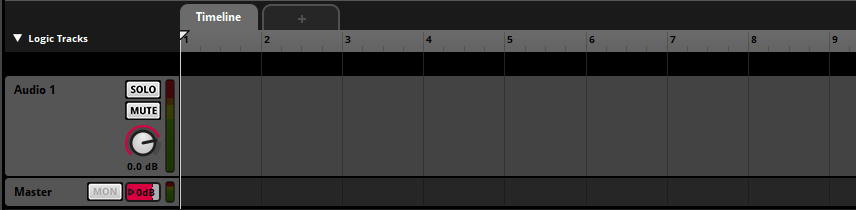
Other parameters are collectively referred to as Game Parameters because they are used to represent the state of the game:

The current value of any parameter can be changed dynamically in the engine. Parameter values can also be controlled by modulators. Each instance of an Event can have different parameter values, so different instances of the same event can deliver a highly diverse listening experience.
The values of the Parameters can be updated using code in the game, but can also be set by automation and adjusted by modulators in the authoring application.
In the example above, you can see that four Parameters were created for the event, each associated with FMOD Studio effects:
- Reverb: Controls the wet value of the reverb effect (0-1)
- Delay: Controls the wet value of the delay effect (0-1)
- DelayTime: Controls the delay time (0-5000ms)
- Pitch: Controls the pitch value of the event (-12st – 12st)
Parameter Types in FMOD Studio
Beginning with FMOD Studio 2.0.0 version, two new parameter types have been added to the existing Continuous Parameters. I will quote FMOD’s glossary documentation to explain briefly how these parameter types work:
Continuous Parameters
Continuous Parameters are a type of parameter that use float numbers. Each parameter has a minimum and maximum value and can be set to any floating point value within this range. Like all parameters, the continuous user parameters can be adjusted to represent any variable in the game project. Continuous parameters are most commonly used for variables that can change in very fine or arbitrary increments.
Discrete Parameters (new)
Discrete Parameters are a type of parameter that use integer (int) numbers. Each discrete parameter has a minimum and maximum value and can be set to any integer value within this range.
Labeled Parameters (new)
Labeled Parameters are a type of parameter that use strings. Each Labeled Parameter has a predefined list of labelled values and can be set to any of these values.
Using the StudioParameterTrigger Node in Godot 4
If we want to change parameters without writing any code, we can use the StudioParameterTrigger node in combination with the StudioEventEmitter3D (or 2D) node:

In this example, the StudioEventEmitter3D node has an Event selected that contains several parameters that will be displayed in Initial Parameter Values property group:
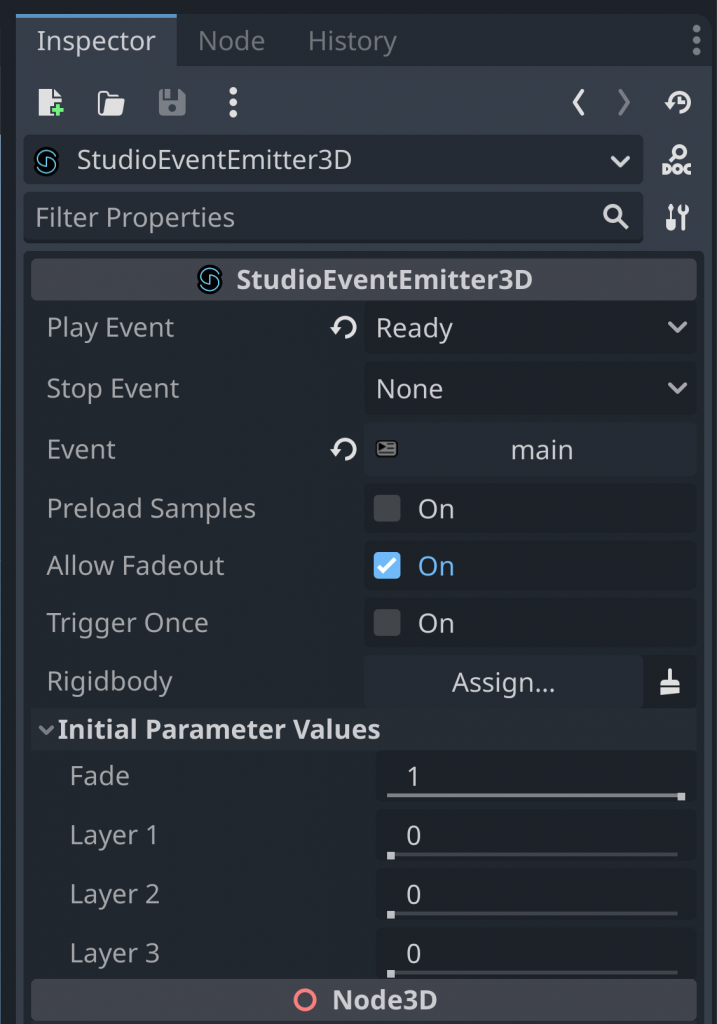
If you now switch to the StudioParameterTrigger node and click on the “Assign” button in the inspector, you can select the StudioEventEmitter node from a new window that will show you the Scene Tree with its nodes:
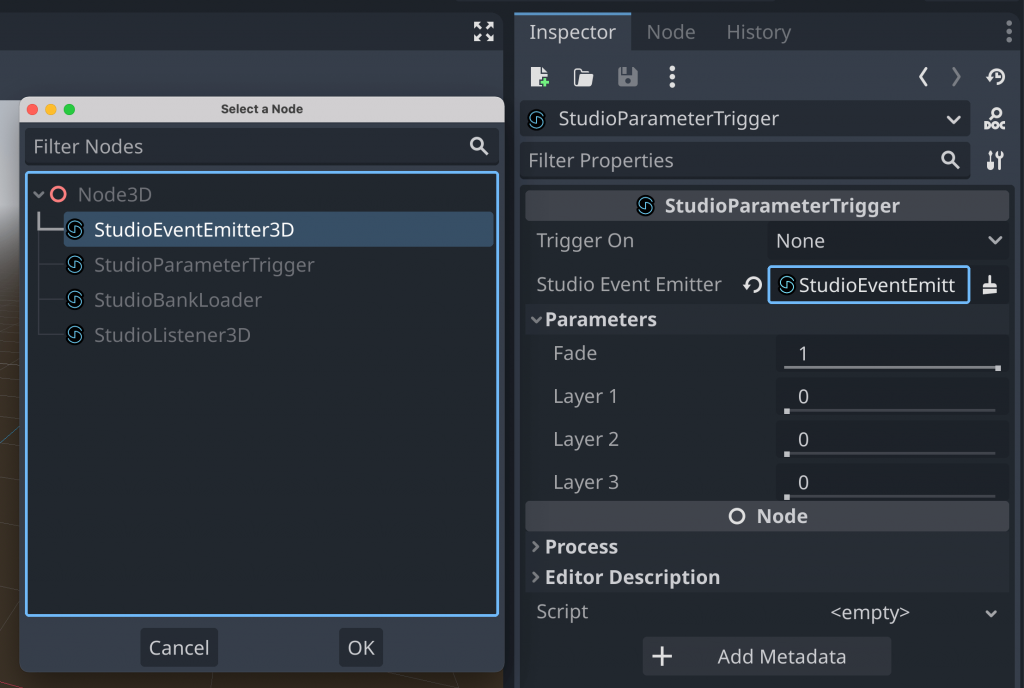
Selecting the Emitter will display the Parameters of the Event in the inspector as you can see the image above. You can decide to trigger parameter changes on Enter Tree, Ready, or Exit Tree. Additionally, if you leave the Trigger On property to None, you can trigger the changes by referencing the StudioParameterTrigger node and calling trigger
on it:
extends Node3D
func _ready():
$StudioParameterTrigger.trigger()
Setting FMOD Parameters with GDScript in Godot
Of course, the whole process is also very simple in GDScript.
In this example script we create an instance of an Event and set the parameter called “end” to the end_parameter
value by calling set_parameter_by_name
on the instance every frame:
extends Node3D
@export var event: EventAsset
var instance: EventInstance
@export_range(0.0, 1.0) var end_parameter: float
func _ready() -> void:
instance = FMODRuntime.create_instance(event)
instance.start()
func _process(delta) -> void::
instance.set_parameter_by_name("end", end_parameter, false)
set_parameter_by_name
takes the name of the parameter, the parameter value and a boolean value that specifies if the seek speed should be ignored when setting the parameter
The @export_range
annotation is helpful to make variables accessible in the inspector. The first value is the minimum value and and second value the maximum value. These two values are represented in the inspector with a slider.
Setting Labeled Parameters in Godot 4
Nowadays you can create Labeled Parameters in FMOD Studio. These Parameters work the same as other Parametery types and you can set them with EventInstance.set_parameter_by_name
. You can find the float values of the Labeled Parameter in the Edit Parameter window in FMOD Studio:
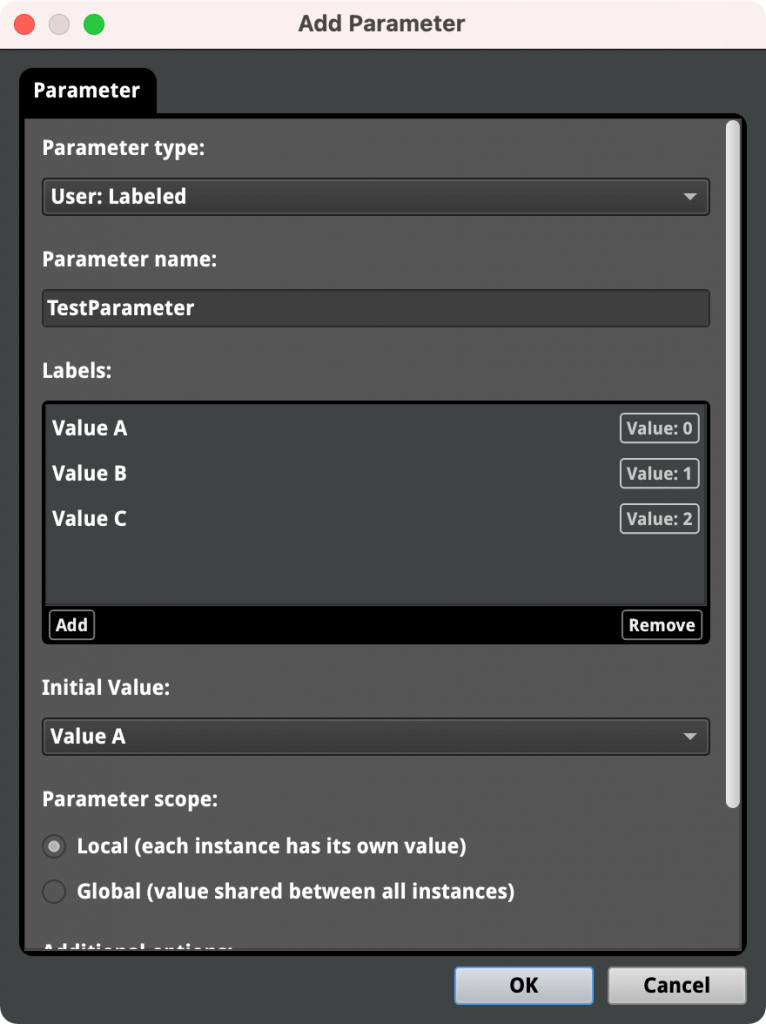
An alternative to using EventInstance.set_parameter_by_name
in this case is to call EventInstance.set_parameter_by_name_with_label
:
extends Node3D
@export var event: EventAsset
var instance: EventInstance
func _ready():
instance = FMODRuntime.create_instance(event)
instance.start()
instance.set_parameter_by_name_with_label("TestParameter", "Value C", false)
Using setParameterByID to control FMOD Parameters in Godot 4
Another way to change parameters in code is to cache the Parameter Id and set the parameter value by id instead of directly passing the parameter name to FMOD. This requires a bit more code but is faster in general when getting or setting parameters multiple times.
To achieve this behaviour, the only addition we need to make is to declare the PARAMETER_ID
variable for each parameter at the start of the class:
extends Node3D
@export var event: EventAsset
var description: EventDescription
var instance: EventInstance
var param_id
func _ready():
description = FMODRuntime.get_event_description(event)
var param_description = description.get_parameter_description_by_name("TestParameter")
param_id = param_description.id
instance = description.create_instance()
instance.start()
instance.set_parameter_by_id_with_label(param_id, "Value C", false)
Setting Global Parameters in FMOD Studio and Godot 4
FMOD Studio supports Global Parameters since version 2.0. Different Events can have the same Parameters and parameter changes affect all EventInstances that contain the Parameter. Previously, you had to set parameters for each single Event. To activate a Global Parameter, set the Parameter scope setting to Global in FMOD Studio during the parameter creation step:
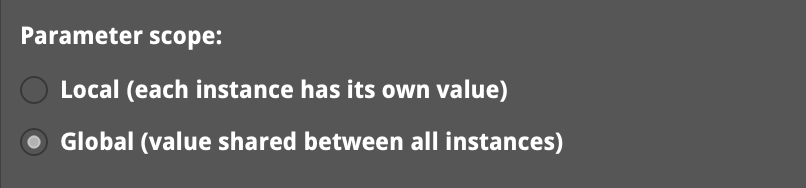
StudioGlobalParameterTrigger Node
You can use the provided StudioGlobalParameterTrigger Node to set a parameter without writing any code. Select the Global Parameter from the a list of parameters in the Parameter property and the parameter will appear direct below:
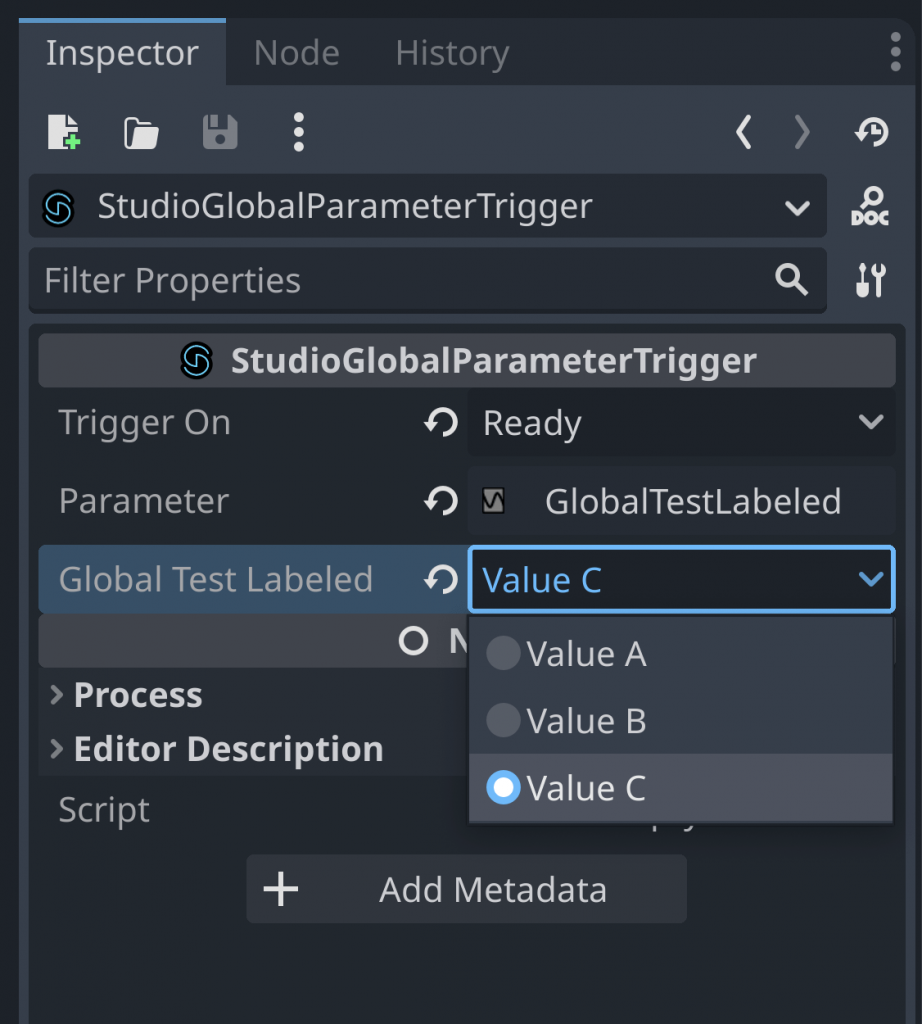
Setting Global Parameters in GDScript
In Godot you need to call set_parameter_by_name
on the StudioSystem
object to set a Global Parameter:
extends Node3D
@export var event: EventAsset
var instance: EventInstance
@export_range(0.0, 1.0) var end_parameter: float
func _ready() -> void:
instance = FMODRuntime.create_instance(event)
instance.start()
func _process(delta) -> void:
FMODStudioModule.get_studio_system().set_parameter_by_name("end", end_parameter, false)
You can access the StudioSystem
instance through the FMODStudioModule
singleton.