The FMOD mixer can be controlled with all its functions in Godot. In this tutorial I’ll show yow how to control the volume of busses, VCAs and trigger snapshots directly from Godot using GDScript.
Bus and VCA volume control in Godot 4
Busses and VCAs in Godot can be controlled in two simple steps:
- Getting an instance of a Bus/VCA
- Adjusting the volume of the Bus/VCA
Let’s take a closer look at these steps by showing an example for Busses:
Getting the Bus
Export a BusAsset and also declare Bus variable in your script:
extends Node3D
@export var bus_asset: BusAsset
var bus: Bus
func _ready():
bus = FMODStudioModule.get_studio_system().get_bus(bus_asset.path)
In the inspector you will be able to select the Bus from a list of Busses:
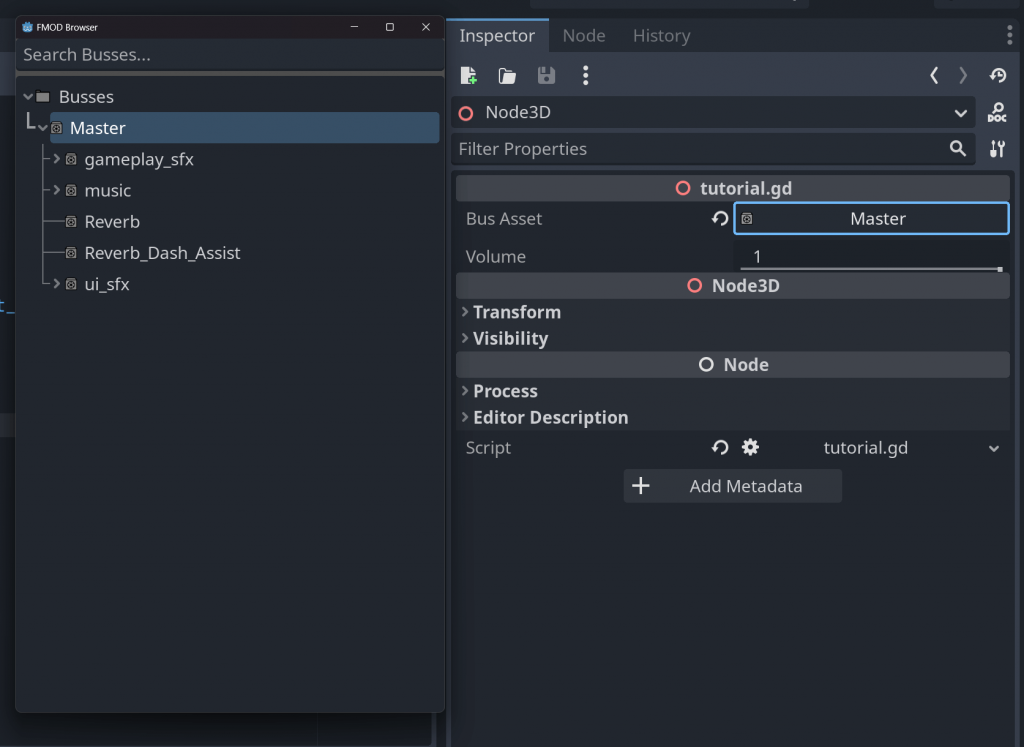
Alternatively, you can use the path of the bus
extends Node3D
var bus_path: String = "bus:/TestBus"
var bus: Bus
func _ready():
bus = FMODStudioModule.get_studio_system().get_bus(bus_path)
If you have generated the GUIDs for your project you can also get the Bus by ID:
extends Node3D
var bus: Bus
func _ready():
bus = FMODStudioModule.get_studio_system().get_bus_by_id(FMODGuids.Busses.MASTER_BUS)
Setting the volume of a Bus
In this example we set our desired volume for the Bus by calling set_volume
on the Bus
instance. set_volume
takes a float value ranging from 0 (mute) to 1 (full volume):
extends Node3D
@export var bus_asset: BusAsset
var bus: Bus
@export_range(0.0, 1.0) var volume: float = 1.0
func _ready():
bus = FMODStudioModule.get_studio_system().get_bus(bus_asset.path)
func _process(delta):
if bus:
bus.set_volume(volume)
Keep in mind that the value set in set_volume
is applied as a scaling factor to the volume level set in FMOD Studio.
Setting the volume of a VCA
Setting the volume of a VCA is very similar to doing it for Busses. Get the VCA
instance using the StudioSystem object and call set_volume
on it:
extends Node3D
@export var vca_asset: VCAAsset
var vca: VCA
@export_range(0.0, 1.0) var volume: float = 1.0
func _ready():
vca = FMODStudioModule.get_studio_system().get_vca(vca_asset.path)
func _process(delta):
if vca:
vca.set_volume(volume)
get_vca_id
is also available in this case.
Triggering FMOD Snapshots in Godot
In FMOD, Snapshots are like normal Events. Triggering snapshots consists in playing them back using the StudioEventEmitter nodes or manually playing Events in code.
This is an example of triggering a Snapshot:
extends Node3D
var event_path = "snapshot:/TestSnapshot"
var event_instance: EventInstance
func _ready():
event_instance = FMODRuntime.create_instance_path(event_path)
event_instance.start()
As you can see here, only the path of the Event changes. Instead of event:/
we now use snapshot:/
to point to the correct path.