If we are planning and organizing our FMOD project in a spreadsheet, we can easily import that spreadsheet file into FMOD Studio and bulk create events for example. Let’s take a look at this minimal spreadsheet example:
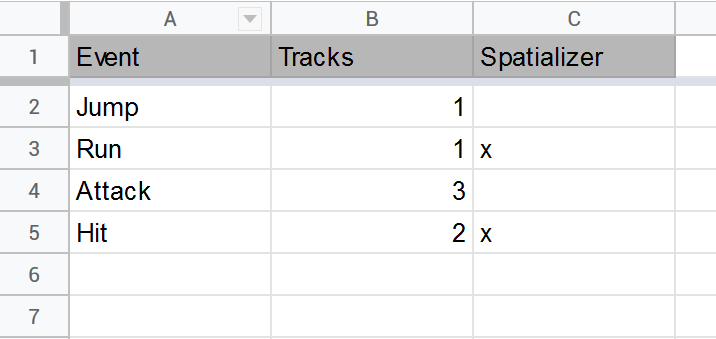
Here we can find three rows. The first Event column will hold the individual event names. The Tracks column specifies how many group tracks will be created inside the event and in the final Spatializer column we can check if we want to add a spatializer effect to the master track of the event.
Creating the .js script to import a .csv file into FMOD Studio
If you haven’t already, create a scripts folder inside your FMOD project or FMOD install directory. Create a new .js file and paste this code inside it:
studio.menu.addMenuItem({
name: "Scripts\\CreateEventsFromCSV",
execute: function () {
function doCSVToEvents(widget) {
var path = widget.findWidget("m_csvFilePath").text();
if (path.substr(path.length - 4).toUpperCase() !== ".CSV") {
studio.system.message("Not a CSV file: " + path);
return;
}
var file = studio.system.getFile(path);
file.open(studio.system.openMode.ReadOnly);
var fileSize = file.size();
if (file.exists()) {
var csv = file.readText(fileSize);
var obj = CSVtoObj(csv)
CreateEvents(obj);
}
}
function CreateEvents(obj) {
for (var i = 0; i < obj.length; i++) {
var myEvent = studio.project.create("Event");
myEvent.name = obj[i].Event;
myEvent.folder = studio.project.workspace.masterEventFolder;
for (var j = 0; j < obj[i].Tracks; j++) {
var track = myEvent.addGroupTrack();
}
if (obj[i].Spatializer == "x") {
myEvent.masterTrack.mixerGroup.effectChain.addEffect('SpatialiserEffect');
}
}
}
function CSVtoObj(csv) {
var arr = csv.split('\n');
var jsonObj = [];
var headers = arr[0].split(',');
for (var i = 1; i < arr.length; i++) {
var data = arr[i].split(',');
var obj = {};
for (var j = 0; j < data.length; j++) {
obj[headers[j].trim()] = data[j].trim();
}
jsonObj.push(obj);
}
return jsonObj;
}
studio.ui.showModalDialog({
windowTitle: "CSV To Events",
windowWidth: 340,
windowHeight: 120,
widgetType: studio.ui.widgetType.Layout,
layout: studio.ui.layoutType.VBoxLayout,
items: [
{
widgetType: studio.ui.widgetType.Label,
widgetId: "m_csvFilePathLabel",
text: "CSV File Path"
},
{
widgetType: studio.ui.widgetType.PathLineEdit,
widgetId: "m_csvFilePath",
text: "*.csv",
pathType: studio.ui.pathType.OpenFile
},
{
widgetType: studio.ui.widgetType.Layout,
layout: studio.ui.layoutType.HBoxLayout,
contentsMargins: {
left: 0,
top: 12,
right: 0,
bottom: 0
},
items: [{
widgetType: studio.ui.widgetType.Spacer,
sizePolicy: {
horizontalPolicy: studio.ui.sizePolicy.MinimumExpanding
}
},
{
widgetType: studio.ui.widgetType.PushButton,
text: "Add Events",
onClicked: function () {
doCSVToEvents(this);
this.closeDialog();
}
}
]
}
]
});
}
});
ui.showModalDialog displays a dialog window in which we can select our desired .csv file when executing the script:
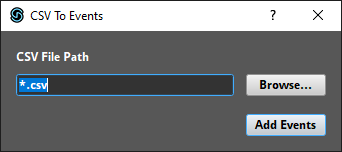
When clicking on Add Events the doCSVToEvents
function will be executed. There we are getting the path of the .csv file, passing the content of the file to the CSVtoObj
function and finally creating the events with the CreateEvents
function.
We create a FMOD Studio Event by calling studio.project.create("Event")
, then we assign the cell content of the Events column to the name property of the event. Based on Tracks value in the spreadsheet we create the correct number of group tracks by calling addGroupTrack()
. Finally, we check if the Spatializer cell contains an x and add a spatializer effect to the master track by calling event.masterTrack.mixerGroup.effectChain.addEffect('SpatialiserEffect')
.
After executing the script the event browser will be populated with events listed in the spreadsheet:

Feel free to modify and expand this example to your personal needs.